Implementing Account Linking
How to implement Pley Connect in your Mobile Game
Pley Connect Implementation Overview
Pley Connect has two ways of initiating account linking between mobile and web. These require minor changes to the web game, the mobile game, and the game backend.
- Input code from mobile game
- QR code from the web
Read about the Pley Connect User Journeys here.
Read about the Pley Connect Technical Concept here.
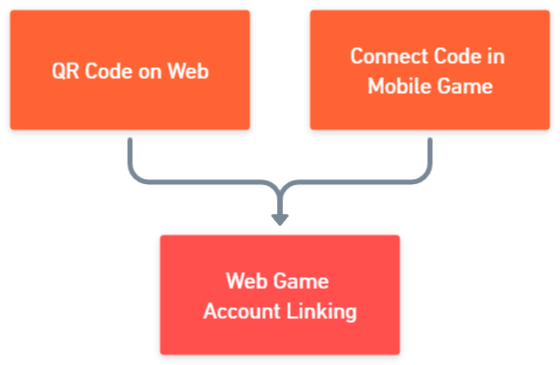
The account linking itself is always executed in the web game, once the accounts are verified by QR or connect code.
Account linking requires:
- Mobile Game: Adding deep linking.
- Mobile Game: Adding a Game Code UI screen.
- Web Game: Adding a link-code check on startup.
- Game Server: Generating and verifying Pley link-codes, saving them to a user.
- Mobile Game, Optional: Adding a Conflict Resolution UI screen.
Implementation QR Code
Read the full QR code deep link implementation here.
1) Mobile App opens through QR code with deeplink game_scheme://pley_account_link?link_code_id=123456
.
2) The game client sends the link_code_id
to the game backend.
3) Game backend verifies the link_code_id with the Pley HTTP API connection-kit/get-link-code
with the payload:
{
"link_code_id": "The linkCodeId from the deep link URL",
"game_id": "Game id that can be found in the Pley Game Manager",
"payload": "user id from the game itself"
}
4) When the game backend receives an OK
from Pley, the backend should respond with an OK
to the game client.
5) If both responses are OK
, the game client will automatically start on the web and the game client should automatically close on mobile.
6) Web game implementation takes over and continues the account linking process.
Implementation Connect Code
Read the full Connect Code UI implementation here.
1) User clicks a button which requests a code
from the game backend.
2) The game backend requests a code from Pley, and returns it to the mobile game.
3) The game displays the code
in the Connect Code UI, along with instructions to visit to pley.com/connect on their desktop.
4) The user inputs the code
on pley.com/connect.
5) Pley verifies the code
and starts the web game for the user.
6) Web game implementation takes over and continues the account linking process.
Implementation Web Game Account Linking
Continues from both Connect Code & QR Code. Read the full web game account linking implementation here.
7) Wait for Pley.SDK.OnInitialized
to be invoked on web game startup.
8) Before authentication with Pley, the game checks if Pley.ConnectionKit.GetAccountLinkCode()
returns a non-empty string. If it exists, pause the loading and send the link_code
to the game backend to verify it.
9) The game backend verifies the link code with Pley using connection-kit/verify-link-code
.
This endpoint returns the Pley game user id
and the payload that was provided to connection-kit/get-link-code
.
{
"game_user_id": "xxxxx-xxxx-xxxx-xxxxxxxxxx", // id of the pley user
"is_guest_user": true/false,
"payload": "user id from the game itself" // payload when code was generated
}
10) The game backend compares the two different game user id:s (Pley user and mobile user), and maps the two users together. The backend should map the user with the least progress to the user with the most progress. Read the account linking guidelines here.
11) When the game client gets a response from the game backend it invokes Pley.ConnectionKit.SetAccountLinkResult
with either Ok
or Failed
. The backend should also set the linking status to true
if successful using connection-kit/set-accountlink-status
.
{
"session_token": "session-token from pley-sdk",
"linked": true
}
12) The game backend responds to the game client that account linking has been completed.
13) The game client calls ConnectionKit.SetAccountLinkResultAsync
so Pley can notify the end user that the linking was successful (documentation / HTTP API).
Enabling Pley Connect on Pley
As a final step, once all parts are implemented, enable Pley connect in the Pley Game Manager at manage.pley.com.
In the Game Manager, under project 'Settings', you'll find Pley Connect.
Add in the information and check to enable Pley Connect. If configured and implemented correctly, it will begin to work instantly.
1) Enable Pley Connect.
2) Add in the game's Deep link scheme (read more here)
3) Add in the Game URL, where the Playable exists.
Find the Game Manager here.
Updated 11 months ago